IO其他流#Properties
目录[ Hide ]
序列流/序列流整合多个
package com.heima.otherio;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.SequenceInputStream;
import java.util.Enumeration;
import java.util.Vector;
public class Demo1_SequenceInputStream {
public static void main(String[] args) throws IOException {
// demo1();
// demo2();
//序列流整合多个
FileInputStream fis1 = new FileInputStream("a.txt");
FileInputStream fis2 = new FileInputStream("b.txt");
FileInputStream fis3 = new FileInputStream("c.txt");
Vector<fileinputstream> v = new Vector<>(); //创建集合对象
v.add(fis1); //将流对象存储进来
v.add(fis2);
v.add(fis3);
Enumeration<fileinputstream> en = v.elements();
SequenceInputStream sis = new SequenceInputStream(en);
FileOutputStream fos = new FileOutputStream("d.txt");
int b ;
while((b = sis.read())!= -1){
fos.write(b);
}
sis.close();
fos.close();
}
private static void demo2() throws FileNotFoundException, IOException {
FileInputStream fis1 = new FileInputStream("a.txt");
FileInputStream fis2 = new FileInputStream("b.txt");
SequenceInputStream sis = new SequenceInputStream(fis1,fis2);
FileOutputStream fos = new FileOutputStream("c.txt");
int b ;
while((b = sis.read())!= -1){
fos.write(b);
}
sis.close();//sis 在关闭的时候,会将构造方法中传入的流对象都关闭
fos.close();
}
private static void demo1() throws FileNotFoundException, IOException {
FileInputStream fis1 = new FileInputStream("a.txt");
FileInputStream fis2 = new FileInputStream("b.txt");
FileOutputStream fos = new FileOutputStream("c.txt");
int b1;
while((b1 = fis1.read()) != -1){
fos.write(b1);
}
fis1.close();
int b2;
while((b2 = fis2.read()) != -1){
fos.write(b2);
}
fis2.close();
fos.close();
}
}
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.SequenceInputStream;
import java.util.Enumeration;
import java.util.Vector;
public class Demo1_SequenceInputStream {
public static void main(String[] args) throws IOException {
// demo1();
// demo2();
//序列流整合多个
FileInputStream fis1 = new FileInputStream("a.txt");
FileInputStream fis2 = new FileInputStream("b.txt");
FileInputStream fis3 = new FileInputStream("c.txt");
Vector<fileinputstream> v = new Vector<>(); //创建集合对象
v.add(fis1); //将流对象存储进来
v.add(fis2);
v.add(fis3);
Enumeration<fileinputstream> en = v.elements();
SequenceInputStream sis = new SequenceInputStream(en);
FileOutputStream fos = new FileOutputStream("d.txt");
int b ;
while((b = sis.read())!= -1){
fos.write(b);
}
sis.close();
fos.close();
}
private static void demo2() throws FileNotFoundException, IOException {
FileInputStream fis1 = new FileInputStream("a.txt");
FileInputStream fis2 = new FileInputStream("b.txt");
SequenceInputStream sis = new SequenceInputStream(fis1,fis2);
FileOutputStream fos = new FileOutputStream("c.txt");
int b ;
while((b = sis.read())!= -1){
fos.write(b);
}
sis.close();//sis 在关闭的时候,会将构造方法中传入的流对象都关闭
fos.close();
}
private static void demo1() throws FileNotFoundException, IOException {
FileInputStream fis1 = new FileInputStream("a.txt");
FileInputStream fis2 = new FileInputStream("b.txt");
FileOutputStream fos = new FileOutputStream("c.txt");
int b1;
while((b1 = fis1.read()) != -1){
fos.write(b1);
}
fis1.close();
int b2;
while((b2 = fis2.read()) != -1){
fos.write(b2);
}
fis2.close();
fos.close();
}
}
内存输出流
package com.heima.otherio;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo2_ByteArrayOutputStream {
public static void main(String[] args) throws IOException {
// demo1();
FileInputStream fis = new FileInputStream("e.txt");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
int b;
while((b = fis.read()) != -1){
baos.write(b);
}
//byte[] arr = baos.toByteArray();//将缓冲区中的所有数据全部取出来,并赋值给arr数组
//System.out.println(new String(arr));
System.out.println(baos.toString());//将缓冲区的内容转换为字符串,再输出语句中可以省略调用ToString方法
fis.close();
}
private static void demo1() throws FileNotFoundException, IOException {
FileInputStream fis = new FileInputStream("e.txt");
byte[] arr = new byte[3];
int len;
while((len = fis.read(arr)) != -1){
System.out.println(new String(arr,0,len));
}
fis.close();
}
}
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo2_ByteArrayOutputStream {
public static void main(String[] args) throws IOException {
// demo1();
FileInputStream fis = new FileInputStream("e.txt");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
int b;
while((b = fis.read()) != -1){
baos.write(b);
}
//byte[] arr = baos.toByteArray();//将缓冲区中的所有数据全部取出来,并赋值给arr数组
//System.out.println(new String(arr));
System.out.println(baos.toString());//将缓冲区的内容转换为字符串,再输出语句中可以省略调用ToString方法
fis.close();
}
private static void demo1() throws FileNotFoundException, IOException {
FileInputStream fis = new FileInputStream("e.txt");
byte[] arr = new byte[3];
int len;
while((len = fis.read(arr)) != -1){
System.out.println(new String(arr,0,len));
}
fis.close();
}
}
面试题😊
package com.heima.test;
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class Test1 {
public static void main(String[] args) throws IOException {
FileInputStream fis = new FileInputStream("a.txt");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] arr = new byte[5];
int len;
while((len = fis.read(arr))!= -1){
baos.write(arr,0,len);
}
System.out.println(baos);
fis.close();
}
}
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class Test1 {
public static void main(String[] args) throws IOException {
FileInputStream fis = new FileInputStream("a.txt");
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] arr = new byte[5];
int len;
while((len = fis.read(arr))!= -1){
baos.write(arr,0,len);
}
System.out.println(baos);
fis.close();
}
}
随机访问流概述和读写数据
package com.heima.otherio;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.RandomAccessFile;
public class Demo8_RandomAccessFile {
public static void main(String[] args) throws IOException {
RandomAccessFile raf = new RandomAccessFile("g.txt", "rw");
//raf.write(97);
// int x = raf.read();
// System.out.println(x);
raf.seek(10);
raf.write(98);
raf.close();
}
}
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.RandomAccessFile;
public class Demo8_RandomAccessFile {
public static void main(String[] args) throws IOException {
RandomAccessFile raf = new RandomAccessFile("g.txt", "rw");
//raf.write(97);
// int x = raf.read();
// System.out.println(x);
raf.seek(10);
raf.write(98);
raf.close();
}
}
对象操作流ObjecOutputStream
package com.heima.otherio;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import com.heima.bean.Person;
public class Demo3_ObjectOutputStream {
/*
* 序列化:将对象写到文件上
* */
public static void main(String[] args) throws IOException, IOException {
Person p1 = new Person("张三",23);
Person p2 = new Person("李四",23);
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("e.txt"));
oos.writeObject(p1);
oos.writeObject(p2);
oos.close();
}
}
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import com.heima.bean.Person;
public class Demo3_ObjectOutputStream {
/*
* 序列化:将对象写到文件上
* */
public static void main(String[] args) throws IOException, IOException {
Person p1 = new Person("张三",23);
Person p2 = new Person("李四",23);
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("e.txt"));
oos.writeObject(p1);
oos.writeObject(p2);
oos.close();
}
}
对象操作流ObjectInputStream
package com.heima.otherio;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.ObjectInputStream;
import com.heima.bean.Person;
public class Demo4_ObjectInputStream {
public static void main(String[] args) throws IOException, IOException, ClassNotFoundException {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("e.txt"));
Person p1 = (Person) ois.readObject();
Person p2 = (Person) ois.readObject();
ois.close();
System.out.println(p1);
System.out.println(p2);
}
}
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.ObjectInputStream;
import com.heima.bean.Person;
public class Demo4_ObjectInputStream {
public static void main(String[] args) throws IOException, IOException, ClassNotFoundException {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("e.txt"));
Person p1 = (Person) ois.readObject();
Person p2 = (Person) ois.readObject();
ois.close();
System.out.println(p1);
System.out.println(p2);
}
}
对象操作流优化
package com.heima.otherio;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.util.ArrayList;
import com.heima.bean.Person;
public class Demo3_ObjectOutputStream {
/*
* 序列化:将对象写到文件上
* */
public static void main(String[] args) throws IOException, IOException {
// demo1();
Person p1 = new Person("张三",23);
Person p2 = new Person("李四",23);
Person p3 = new Person("王五",23);
Person p4 = new Person("赵六",23);
ArrayList<person> list = new ArrayList<>();
list.add(p1);
list.add(p2);
list.add(p3);
list.add(p4);
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("e.txt"));
oos.writeObject(list);
oos.close();
}
private static void demo1() throws IOException, FileNotFoundException {
Person p1 = new Person("张三",23);
Person p2 = new Person("李四",23);
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("e.txt"));
oos.writeObject(p1);
oos.writeObject(p2);
oos.close();
}
}
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.util.ArrayList;
import com.heima.bean.Person;
public class Demo3_ObjectOutputStream {
/*
* 序列化:将对象写到文件上
* */
public static void main(String[] args) throws IOException, IOException {
// demo1();
Person p1 = new Person("张三",23);
Person p2 = new Person("李四",23);
Person p3 = new Person("王五",23);
Person p4 = new Person("赵六",23);
ArrayList<person> list = new ArrayList<>();
list.add(p1);
list.add(p2);
list.add(p3);
list.add(p4);
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("e.txt"));
oos.writeObject(list);
oos.close();
}
private static void demo1() throws IOException, FileNotFoundException {
Person p1 = new Person("张三",23);
Person p2 = new Person("李四",23);
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("e.txt"));
oos.writeObject(p1);
oos.writeObject(p2);
oos.close();
}
}
package com.heima.otherio;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.util.ArrayList;
import com.heima.bean.Person;
public class Demo4_ObjectInputStream {
public static void main(String[] args) throws IOException, IOException, ClassNotFoundException {
// demo1();
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("e.txt"));
ArrayList<Person> list = (ArrayList<Person>) ois.readObject();//将集合对象一次读取
for (Person person : list) {
System.out.println(person);
}
ois.close();
}
private static void demo1() throws IOException, FileNotFoundException, ClassNotFoundException {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("e.txt"));
Person p1 = (Person) ois.readObject();
Person p2 = (Person) ois.readObject();
ois.close();
System.out.println(p1);
System.out.println(p2);
}
}
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.util.ArrayList;
import com.heima.bean.Person;
public class Demo4_ObjectInputStream {
public static void main(String[] args) throws IOException, IOException, ClassNotFoundException {
// demo1();
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("e.txt"));
ArrayList<Person> list = (ArrayList<Person>) ois.readObject();//将集合对象一次读取
for (Person person : list) {
System.out.println(person);
}
ois.close();
}
private static void demo1() throws IOException, FileNotFoundException, ClassNotFoundException {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("e.txt"));
Person p1 = (Person) ois.readObject();
Person p2 = (Person) ois.readObject();
ois.close();
System.out.println(p1);
System.out.println(p2);
}
}
加上id号
为了区别当前改版是第几版 不加也可以
package com.heima.bean;
import java.io.Serializable;
public class Person implements Serializable{
/**
*
*/
private static final long serialVersionUID = 2L;
private String name;
private int age;
public Person() {
super();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Person(String name, int age) {
super();
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Person [name=" + name + ", age=" + age + "]";
}
}
import java.io.Serializable;
public class Person implements Serializable{
/**
*
*/
private static final long serialVersionUID = 2L;
private String name;
private int age;
public Person() {
super();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Person(String name, int age) {
super();
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Person [name=" + name + ", age=" + age + "]";
}
}
数据输入输出流
package com.heima.otherio;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo9_Data {
public static void main(String[] args) throws IOException {
//demo1();
// demo2();
// demo3();
DataInputStream dis = new DataInputStream(new FileInputStream("h.txt"));
int x = dis.readInt();
int y = dis.readInt();
int z = dis.readInt();
System.out.println(x);
System.out.println(y);
System.out.println(z);
dis.close();
}
private static void demo3() throws FileNotFoundException, IOException {
DataOutputStream dos = new DataOutputStream(new FileOutputStream("h.txt"));
dos.writeInt(997);
dos.writeInt(998);
dos.writeInt(999);
dos.close();
}
private static void demo2() throws FileNotFoundException, IOException {
FileInputStream fis = new FileInputStream("h.txt");
int x = fis.read();
int y = fis.read();
int z = fis.read();
System.out.println(x);
System.out.println(y);
System.out.println(z);
fis.close();
}
private static void demo1() throws FileNotFoundException, IOException {
FileOutputStream fos = new FileOutputStream("h.txt");
fos.write(997);
fos.write(998);
fos.write(999);
fos.close();
}
}
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo9_Data {
public static void main(String[] args) throws IOException {
//demo1();
// demo2();
// demo3();
DataInputStream dis = new DataInputStream(new FileInputStream("h.txt"));
int x = dis.readInt();
int y = dis.readInt();
int z = dis.readInt();
System.out.println(x);
System.out.println(y);
System.out.println(z);
dis.close();
}
private static void demo3() throws FileNotFoundException, IOException {
DataOutputStream dos = new DataOutputStream(new FileOutputStream("h.txt"));
dos.writeInt(997);
dos.writeInt(998);
dos.writeInt(999);
dos.close();
}
private static void demo2() throws FileNotFoundException, IOException {
FileInputStream fis = new FileInputStream("h.txt");
int x = fis.read();
int y = fis.read();
int z = fis.read();
System.out.println(x);
System.out.println(y);
System.out.println(z);
fis.close();
}
private static void demo1() throws FileNotFoundException, IOException {
FileOutputStream fos = new FileOutputStream("h.txt");
fos.write(997);
fos.write(998);
fos.write(999);
fos.close();
}
}
打印流的概述和特点

package com.heima.otherio;
import com.heima.bean.Person;
import javax.swing.plaf.synth.SynthOptionPaneUI;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintStream;
import java.io.PrintWriter;
/**
* Code by pureqh on 2021-02-25
*/
public class Demo5_PrintStream {
/*
* PrintStream和PrintWriteer分别是打印的字节流和字符流
* */
public static void main(String[] args) throws FileNotFoundException {
//demo1();
PrintWriter pw = new PrintWriter(new FileOutputStream("f.txt"),true);
pw.println(97); //自动刷出功能 只 针 对 println方法
pw.print(97);
pw.write(97);
//pw.close();
}
private static void demo1() {
System.out.println();
PrintStream ps = System.out; //获取标准输出流
ps.println("111"); //底层通过tostring将97抓换成字符串打印
ps.write(97); //查找码表找到对应的a打印
Person p1 = new Person("李四",23);
ps.println(p1); //默认调用p1的tostring方法
Person p2 = null; //打印引用数据类型,如果是null,就打印null,如果不是null就打印对象的tostring方法
ps.println(p2);
ps.close();
}
}
import com.heima.bean.Person;
import javax.swing.plaf.synth.SynthOptionPaneUI;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintStream;
import java.io.PrintWriter;
/**
* Code by pureqh on 2021-02-25
*/
public class Demo5_PrintStream {
/*
* PrintStream和PrintWriteer分别是打印的字节流和字符流
* */
public static void main(String[] args) throws FileNotFoundException {
//demo1();
PrintWriter pw = new PrintWriter(new FileOutputStream("f.txt"),true);
pw.println(97); //自动刷出功能 只 针 对 println方法
pw.print(97);
pw.write(97);
//pw.close();
}
private static void demo1() {
System.out.println();
PrintStream ps = System.out; //获取标准输出流
ps.println("111"); //底层通过tostring将97抓换成字符串打印
ps.write(97); //查找码表找到对应的a打印
Person p1 = new Person("李四",23);
ps.println(p1); //默认调用p1的tostring方法
Person p2 = null; //打印引用数据类型,如果是null,就打印null,如果不是null就打印对象的tostring方法
ps.println(p2);
ps.close();
}
}
标准输入输出流概述和输出语句
package com.heima.otherio;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintStream;
/**
* Code by pureqh on 2021-02-26
*/
public class Demo6_SystemInOut {
public static void main(String[] args) throws IOException {
//demo1();
System.setIn(new FileInputStream("a.txt")); //改变标准输入流
System.setOut(new PrintStream("b.txt")); //改变标准输出流
InputStream is = System.in; //获取标准的键盘输入流,默认指向键盘,改变后指向文件
PrintStream ps = System.out; //获取标准输出流,默认指向控制台,改变后指向文件
int b;
while ((b = is.read()) != -1){
ps.write(b);
}
//System.out.println(); 也是一个输出流 不需要关流 指向的是控制台
is.close();
ps.close();
}
private static void demo1() throws IOException {
InputStream is = System.in;
int x = is.read();
System.out.println(x);
is.close();
}
}
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.PrintStream;
/**
* Code by pureqh on 2021-02-26
*/
public class Demo6_SystemInOut {
public static void main(String[] args) throws IOException {
//demo1();
System.setIn(new FileInputStream("a.txt")); //改变标准输入流
System.setOut(new PrintStream("b.txt")); //改变标准输出流
InputStream is = System.in; //获取标准的键盘输入流,默认指向键盘,改变后指向文件
PrintStream ps = System.out; //获取标准输出流,默认指向控制台,改变后指向文件
int b;
while ((b = is.read()) != -1){
ps.write(b);
}
//System.out.println(); 也是一个输出流 不需要关流 指向的是控制台
is.close();
ps.close();
}
private static void demo1() throws IOException {
InputStream is = System.in;
int x = is.read();
System.out.println(x);
is.close();
}
}
两种方式实现键盘录入
package com.heima.otherio;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Scanner;
/**
* Code by pureqh on 2021-02-26
*/
public class Demo7_SystemIn {
public static void main(String[] args) throws IOException {
// BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); //InputStreamreader转换流
// String line = br.readLine();
// System.out.println(line);
// br.close();
Scanner sc = new Scanner(System.in);
String li = sc.nextLine();
System.out.println(li);
sc.close();
}
}
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Scanner;
/**
* Code by pureqh on 2021-02-26
*/
public class Demo7_SystemIn {
public static void main(String[] args) throws IOException {
// BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); //InputStreamreader转换流
// String line = br.readLine();
// System.out.println(line);
// br.close();
Scanner sc = new Scanner(System.in);
String li = sc.nextLine();
System.out.println(li);
sc.close();
}
}
Properties的概述和作为Map集合的使用,特殊功能使用
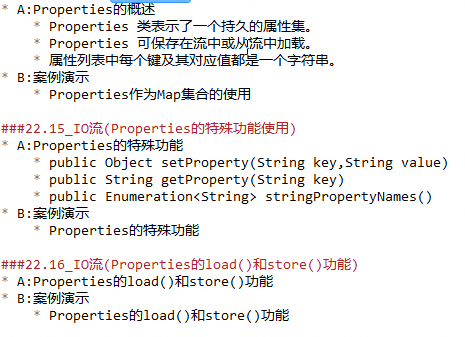
package com.heima.otherio;
import com.sun.org.apache.bcel.internal.generic.POP;
import java.util.Enumeration;
import java.util.Properties;
/**
* Code by pureqh on 2021-02-26
*/
public class Demo10_Properties {
public static void main(String[] args) {
//demo1();
Properties prop = new Properties();
prop.setProperty("name","张三");
prop.setProperty("tel","18912345678");
// System.out.println(prop);
Enumeration<String> en = (Enumeration<String>) prop.propertyNames();
while (en.hasMoreElements()){
String key = en.nextElement(); //获取propertyNames中的每一个键
String value = prop.getProperty(key); //根据键获取值
System.out.println(key + "=" +value);
}
}
private static void demo1() {
Properties prop = new Properties();
prop.put("abc",123);
System.out.println(prop);
}
}
import com.sun.org.apache.bcel.internal.generic.POP;
import java.util.Enumeration;
import java.util.Properties;
/**
* Code by pureqh on 2021-02-26
*/
public class Demo10_Properties {
public static void main(String[] args) {
//demo1();
Properties prop = new Properties();
prop.setProperty("name","张三");
prop.setProperty("tel","18912345678");
// System.out.println(prop);
Enumeration<String> en = (Enumeration<String>) prop.propertyNames();
while (en.hasMoreElements()){
String key = en.nextElement(); //获取propertyNames中的每一个键
String value = prop.getProperty(key); //根据键获取值
System.out.println(key + "=" +value);
}
}
private static void demo1() {
Properties prop = new Properties();
prop.put("abc",123);
System.out.println(prop);
}
}